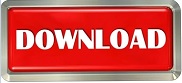
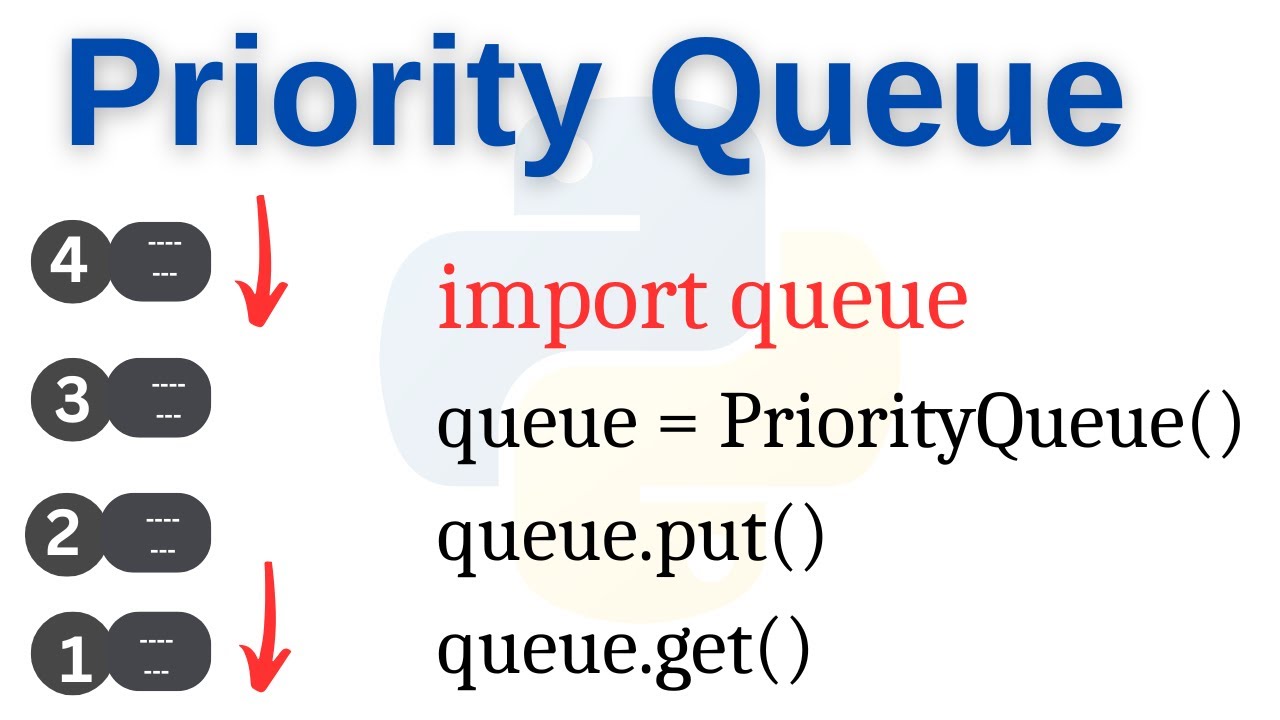
But again, if two elements are of the same value, then the order is taken into consideration while removing the same elements from the Python queue. import queueĪs we can see here, the item with the lowest value gets out first. We will be using the q.qsize() function (returns the size of the queue) in order to run the for loop function. Now that we have the items in the queue, let us use the Python for loop to remove the items. Let us add 5 elements into a queue using the priority queue function. Let us see how this works with the help of an example. But how is that going to work? Well, the logic behind this policy is that the lowest-valued item gets out first.Īgain, to be able to perform priority queue operations, we have to use another function shown below. What really matters is the value of the items. Here, the order in which we put the items doesn’t matter. The priority queue in Python is a bit interesting. You can run this script to interact with the queue by adding, removing, and displaying elements based on the user’s input. The loop continues until the user chooses to quit. The main program loop allows users to choose between adding an element, removing an element, displaying the elements, or quitting the program. The display_queue() function iterates through the elements in the queue list and displays them. The dequeue() function removes the first element from the queue using the pop(0) method.

In this script, the enqueue() function adds an element to the queue by appending it to the end of the queue list. Print(“Element”, element, “removed from the queue.”)Įlement = input(“Enter the element to add: “) Print(“Element”, element, “added to the queue.”) Let us explore the LIFO queue with the help of an example: Adding and removing items into a queue: But unlike FIFO (which is considered as the default queue type), we have to add a special function to operate LIFO queues. In other words, the one that goes, at last, comes out first. The script continues to present the menu options to the user until they choose to exit.Īgain, as the name says, in this type of queue, the element that goes in first comes out last. Overall, this script creates a menu-driven interface where the user can add elements to a queue, remove elements from the queue, display the elements in the queue, or exit the program. If the user enters an invalid choice (other than `’1’`, `’2’`, `’3’`, or `’4’`), we display a message indicating that the choice is invalid, and the loop continues to prompt the user for another choice. If the user enters `’4’`, we display a message indicating that the program is exiting, and then we break out of the loop, ending the program. If the queue is empty, we display a message indicating that the queue is empty. If the queue is not empty, we print all the elements in the queue using a `for` loop. If the user enters `’3’`, we again check if the queue is not empty (`len(queue) > 0`). If the queue is not empty, we use the `popleft()` method to remove the element at the front of the queue, and we print the removed element. If the user enters `’2’`, we first check if the queue is not empty (`len(queue) > 0`). Print(“Removed element:”, removed_element) We display a message indicating that the element has been added to the queue. The element is then appended to the end of the queue using the `append()` method. If the user enters `’1’`, we prompt them to enter an element to add to the queue using the `input()` function. Perform the corresponding action based on the user’s choice:Įlement = input(“Enter an element to add: “).The user’s input is stored in the `choice` variable as a string. We use the `input()` function to prompt the user to enter their choice. We print the menu options to the console, presenting the available actions to the user.Ĭhoice = input(“Enter your choice (1-4): “) We use an infinite `while` loop, which means the loop will keep running until we explicitly break out of it. This creates an empty queue object that we will use to store the elements. We create an empty queue using the `deque()` constructor from the `deque` class. The `deque` class provides an implementation of a double-ended queue, which we will use to create a queue data structure. Here, we import the `deque` class from the `collections` module.
#Get priority queue python code
Let’s go through the code step by step to understand how the menu-driven script works:
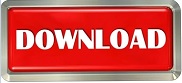